일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | 6 | |
7 | 8 | 9 | 10 | 11 | 12 | 13 |
14 | 15 | 16 | 17 | 18 | 19 | 20 |
21 | 22 | 23 | 24 | 25 | 26 | 27 |
28 | 29 | 30 | 31 |
- Python
- mllib
- Transformer
- 부스트캠프 ai tech 준비과정
- AI 엔지니어 기초 다지기
- TensorFlow
- 부스트클래스
- pycharm
- Spark MLlib
- DataSet
- kaggle
- yolo
- 위기의코딩맨
- 서울 맛집
- AI Tech 준비과정
- 연남 맛집
- NLP
- 맛집
- 자연어
- 캐글
- r
- 데이터 시각화
- 연남동 맛집
- spark
- RDD
- 홍대 맛집
- Ai
- AI tech
- 부스트캠프
- tensorflow 예제
- Today
- Total
We-Co
[We-Co] Softmax regression MNIST 예제 - Tensorflow 본문
안녕하세요. 위기의코딩맨입니다.
오늘은 지금까지 알아본 지식들로 Tensorflow의 가장 기본적인 예제 MNIST 예제를 풀어보도록 하겠습니다.
그리고 임의로 숫자를 넣어서 해당 숫자를 잘 인식하는지 알아보도록 하겠습니다.
[ MNIST ]
먼저 사용할 Tensorflow를 임포트 해줍니다.
import tensorflow as tf
먼저 MNIST에 필요한 데이터를 tf.keras.datasets.mnist.load_data()를 통해서 다운받아서 넣어줍니다.
x_train, x_test의 타입을 float32 타입으로 변경하고, 해당 28*28의 형태를 갖고 있는 이미지를
784차원으로 Flattening 작업을 진행합니다.
그리고 x_tain, y_test의 [0,255]사이의 값들을 [0, 1]로 변경하기위해 255로 나누어 주도록합니다.
마지막으로 해당 데이터를 one-hot Encoding을 적용합니다.
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
x_train, x_test = x_train.astype('float32'), x_test.astype('float32')
x_train, x_test = x_train.reshape([-1, 784]), x_test.reshape([-1, 784])
x_train, x_test = x_train / 255., x_test / 255.
y_train, y_test = tf.one_hot(y_train, depth=10), tf.one_hot(y_test, depth=10)
tf.data API를 이용하여 60000개의 데이터를 섞고 100개의 배치를 적용합니다.
train_data = tf.data.Dataset.from_tensor_slices((x_train, y_train))
train_data = train_data.repeat().shuffle(60000).batch(100)
train_data_iter = iter(train_data)
Softmax Regression 모델을 정의하고
class SoftmaxRegression(tf.keras.Model):
def __init__(self):
super(SoftmaxRegression, self).__init__()
self.softmax_layer = tf.keras.layers.Dense(10,
activation=None,
kernel_initializer='zeros',
bias_initializer='zeros')
def call(self, x):
logits = self.softmax_layer(x)
return tf.nn.softmax(logits)
최적화를 위한 optimizer을 선언해주고
확률적 경사 하강법 (Stochastic Gradient Descent : SGD)
Learning Rate는 0.5로 주도록합니다.
optimizer = tf.optimizers.SGD(0.5)
크로스 엔트로피 손실함수도 정의해주도록 합니다.
def Cross_Entropy_Loss(y_pred, y):
return tf.reduce_mean(-tf.reduce_sum(y * tf.math.log(y_pred), axis=[1]))
최적화에 필요한 Function을 정의하고
def train_step(model, x, y):
with tf.GradientTape() as tape:
y_pred = model(x)
loss = cross_entropy_loss(y_pred, y)
gradients = tape.gradient(loss, model.trainable_variables)
optimizer.apply_gradients(zip(gradients, model.trainable_variables))
모델의 정확도를 출력하는 함수를 정의합니다.
def Compute_Accuracy(y_pred, y):
correct_prediction = tf.equal(tf.argmax(y_pred,1), tf.argmax(y,1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
return accuracy
SoftmaxRegressin Model을 선언해주도록 합니다.
SoftmaxRegression_model = SoftmaxRegression()
1000번의 학습을 진행하도록 합니다.
for i inrange(1000):
batch_xs, batch_ys = next(train_data_iter)
train_step(SoftmaxRegression_model ,batch_xs, batch_ys)
print("정확도 : %f" % compute_accuracy(SoftmaxRegression_model(x_test), y_test))
결과는 91~92%정도의 정확도를 나타내는 작업이 확인됩니다.
from PIL import Image
im = Image.open('/content/T.png')
im = np.array(im)
plt.imshow(im)
im = im.reshape([-1, 784])
im = im / 255.
ima = SoftmaxRegression_model.predict(im)
max(ima[0])
0.929225
list(ima[0]).index(max(ima[0]))
3
학습된 모델을 통해서 제가 임의로 3의 이미지를 만들어서
적용해보고 92%정도의 정확도로 3이라는 값을 받아오는 것을 확인할 수 있었습니다.여기서 학습을 2천번으로 늘려서 진행했습니다.
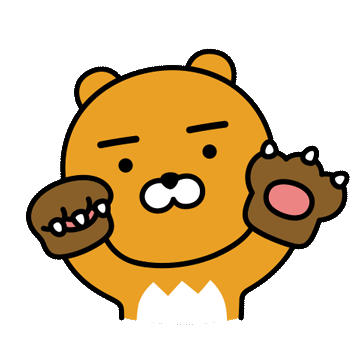
'Python > Tensorflow' 카테고리의 다른 글
[We-Co] CNN의 풀링, 컨볼루션 - Tensorflow (0) | 2021.10.04 |
---|---|
[We-Co] Bidirectional RNN 예제 - MDB Movie Review (0) | 2021.10.02 |
[We-Co] Softmax Regression, Cross-Entropy, One-Hot-Encoding - TensorFlow (0) | 2021.09.27 |
[We-Co] 선형회귀 숫자 예측 - TensorFlow Linear Regression (0) | 2021.09.27 |
[We-Co] TensorFlow 변수,상수선언과 Session() (0) | 2021.07.22 |